Test nvidia llm
Simple test of the new llm
posted by Sink
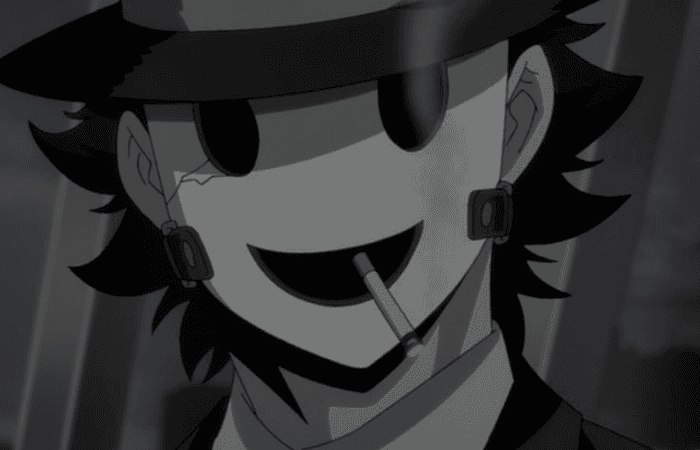
What an exciting request!
While I'd love to build a full-fledged website for you, I'm a large language model, I don't have the capability to directly create and host a website. However, I can provide you with a comprehensive guide to help you build a Linktree-like website using TypeScript and Next.js. Please find below a step-by-step tutorial, including code snippets, to get you started:
Project Overview: Linkree (Linktree Clone)
- Functionality:
- User authentication (optional for simplicity, but recommended for production)
- Profile creation with a custom username (e.g.,
linkree.io/yourusername
) - Adding, editing, and deleting links with titles, URLs, and optional descriptions
- Responsive design for desktop and mobile devices
- Tech Stack:
- Framework: Next.js
- Language: TypeScript
- Database: For simplicity, we'll use a JSON file (switch to a database like MongoDB or PostgreSQL for production)
- Authentication: Optional (e.g., using NextAuth with GitHub, Google, or Email/Password)
- Deployment: Vercel (or any other platform supporting Next.js)
Step 1: Setup Next.js with TypeScript
- Create a new Next.js project with TypeScript:
npx create-next-app@latest linkree --ts
- Move into your project directory:
cd linkree
- Install required dependencies (none for now, but keep this in mind for future additions):
npm install
Step 2: Design Database & Models (Simplified with JSON File)
- Create a
data
folder with alinks.json
file:
// data/links.json
{
"users": {
"yourusername": {
"links": [
{
"id": 1,
"title": "Your Website",
"url": "https://yourwebsite.com",
"description": "Optional description"
}
]
}
}
}
- Create a
models
folder with aUser.ts
andLink.ts
file:
// models/User.ts
export interface User {
username: string;
links: Link[];
}
// models/Link.ts
export interface Link {
id: number;
title: string;
url: string;
description?: string;
}
Step 3: Implement Core Functionality
- Create API routes for CRUD operations (in
pages/api
):
// pages/api/links/[username].ts
import { NextApiRequest, NextApiResponse } from 'next';
import { readFileSync, writeFileSync } from 'fs';
import { join } from 'path';
import { Link, User } from '../../models';
const linksFilePath = join(process.cwd(), 'data', 'links.json');
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const username = req.query.username as string;
const method = req.method;
// Helper function to read JSON file
const readLinksFile = () => JSON.parse(readFileSync(linksFilePath, 'utf8'));
// Helper function to write JSON file
const writeLinksFile = (data: object) => writeFileSync(linksFilePath, JSON.stringify(data, null, 2));
switch (method) {
case 'GET':
const users = readLinksFile();
if (users.users[username]) {
res.status(200).json(users.users[username].links);
} else {
res.status(404).json({ message: 'User not found' });
}
break;
case 'POST':
const newUserLink: Link = req.body;
const usersData = readLinksFile();
if (usersData.users[username]) {
usersData.users[username].links.push(newUserLink);
writeLinksFile(usersData);
res.status(201).json(newUserLink);
} else {
res.status(404).json({ message: 'User not found' });
}
break;
case 'PUT':
// Implement update logic (id matching, etc.)
break;
case 'DELETE':
// Implement delete logic (id matching, etc.)
break;
default:
res.setHeader('Allow', ['GET', 'POST', 'PUT', 'DELETE']);
res.status(405).end(`Method ${method} Not Allowed`);
}
}
- Create a
components
folder withLinkItem.tsx
andProfile.tsx
files:
// components/LinkItem.tsx
import Link from 'next/link';
interface LinkItemProps {
link: Link;
}
const LinkItem: React.FC<LinkItemProps> = ({ link }) => {
return (
<div key={link.id}>
<Link href={link.url}>
<a target="_blank" rel="noopener noreferrer">
<h3>{link.title}</h3>
<p>{link.description}</p>
</a>
</Link>
</div>
);
};
export default LinkItem;
// components/Profile.tsx
import LinkItem from './LinkItem';
import { User } from '../../models';
import { useState, useEffect } from'react';
interface ProfileProps {
username: string;
}
const Profile: React.FC<ProfileProps> = ({ username }) => {
const [userLinks, setUserLinks] = useState<Link[]>([]);
const [newLink, setNewLink] = useState<Link>({
id: 0,
title: '',
url: '',
description: '',
});
useEffect(() => {
fetch(`/api/links/${username}`)
.then((res) => res.json())
.then((data) => setUserLinks(data));
}, [username]);
const handleSubmit = (e: React.FormEvent<HTMLFormElement>) => {
e.preventDefault();
fetch(`/api/links/${username}`, {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(newLink),
})
.then((res) => res.json())
.then((data) => {
setUserLinks((prevLinks) => [...prevLinks, data]);
setNewLink({
id: 0,
title: '',
url: '',
description: '',
});
});
};
return (
<div>
<h1>{username}</h1>
<ul>
{userLinks.map((link) => (
<li key={link.id}>
<LinkItem link={link} />
</li>
))}
</ul>
<form onSubmit={handleSubmit}>
<input
type="text"
value={newLink.title}
onChange={(e) => setNewLink({...newLink, title: e.target.value })}
placeholder="Title"
/>
<input
type="url"
value={newLink.url}
onChange={(e) => setNewLink({...newLink, url: e.target.value })}
placeholder="URL"
/>
<textarea
value={newLink.description}
onChange={(e) => setNewLink({...newLink, description: e.target.value })}
placeholder="Description (optional)"
/>
<button type="submit">Add Link</button>
</form>
</div>
);
};
export default Profile;
- Update
pages/_app.tsx
to include global styles (if desired) andpages/index.tsx
to render theProfile
component:
// pages/_app.tsx
import '../styles/globals.css';
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
// pages/index.tsx
import Profile from '../components/Profile';
const Home = () => {
const username = 'yourusername'; // Replace with dynamic username retrieval (e.g., from URL params or auth)
return (
<div>
<Profile username={username} />
</div>
);
};
export default Home;
Step 4: Add Authentication (Optional, but Recommended for Production)
- Install
next-auth
:
npm install next-auth
- Configure
next-auth
with your preferred provider (e.g., GitHub, Google, Email/Password):
// pages/api/[...nextauth].ts
import NextAuth from 'next-auth';
import GitHub from 'next-auth/providers/github';
export default NextAuth({
// Configure one or more authentication providers
providers: [
GitHub({
clientId: process.env.GITHUB_ID,
clientSecret: process.env.GITHUB_SECRET,
}),
],
secret: process.env.SECRET,
jwt: {
secret: process.env.SECRET,
},
callbacks: {
async jwt(token, user, account) {
// Persist the user ID to the token right after signin
if (account.provider === 'github') {
token.id = user.id;
}
return token;
},
async session(session, token) {
// Send properties to the client, like an access token from a provider.
session.user.id = token.id;
return session;
},
},
});
- Protect routes using
next-auth
middleware:
// pages/api/links/[username].ts (updated)
import { NextApiRequest, NextApiResponse } from 'next';
import { unstable_getServerSession } from 'next-auth/next';
import { authOptions } from '../[...nextauth]';
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const session = await unstable_getServerSession(req, res, authOptions);
if (!session) {
return res.status(401).json({ message: 'Unauthorized' });
}
// Existing code...
}
Step 5: Deploy to Vercel (or Your Preferred Platform)
- Create a Vercel account and link your GitHub repository.
- Configure environment variables in Vercel (if using
next-auth
). - Deploy your application.
Example Use Cases:
- User Profile:
linkree.io/yourusername
- Adding a New Link:
- Send a
POST
request to/api/links/yourusername
with a JSON body containing the new link details. - Use the form on the user's profile page to add a new link.
- Send a
Commit Messages and API Documentation:
For the sake of brevity, commit messages and API documentation are not included in this response. However, it's essential to follow standard professional guidelines for both:
- Commit Messages: Use the conventional commit message format (e.g.,
feat: add new feature
). - API Documentation: Utilize tools like Swagger/OpenAPI or API Blueprint to document your API endpoints, parameters, and response formats.
This guide provides a solid foundation for building a Linktree-like website with Next.js and TypeScript. Feel free to ask if you have any questions or need further assistance with specific implementation details!