LeetCode problems in C#
Detailed post about common techniques to solving Leetcode problems with C#
posted by Sink
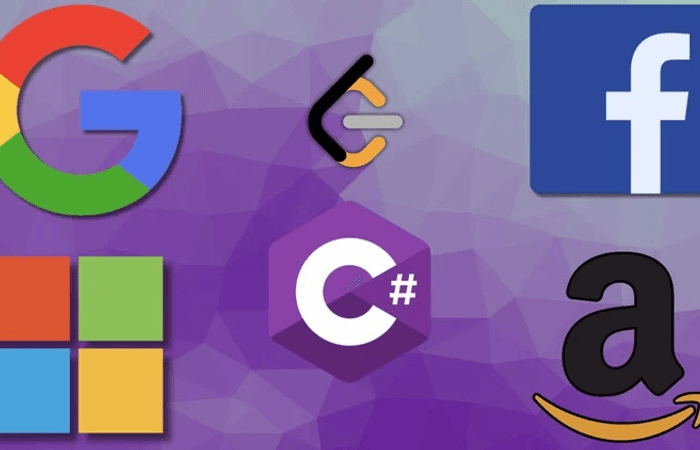
Below is a comprehensive list of concepts, syntax, and techniques necessary for solving LeetCode problems in C#. This includes data structures, algorithms, and popular C# syntax and libraries.
1. Basic C# Syntax
- Variables and Data Types:
int x = 10; double y = 3.14; string name = "Alice"; bool isTrue = true;
- Control Structures:
if (condition) { ... } else if (condition) { ... } else { ... } for (int i = 0; i < 10; i++) { ... } while (condition) { ... } do { ... } while (condition);
- Functions:
public int Add(int a, int b) { return a + b; }
- Classes and Objects:
public class Person { public string Name { get; set; } public int Age { get; set; } public Person(string name, int age) { Name = name; Age = age; } }
2. Data Structures
- Arrays:
int[] arr = new int[5]; int[] arr = { 1, 2, 3, 4, 5 };
- Lists:
List<int> list = new List<int>(); list.Add(1); list.Remove(1); list.Count;
- Stacks:
Stack<int> stack = new Stack<int>(); stack.Push(1); int top = stack.Pop(); int peek = stack.Peek();
- Queues:
Queue<int> queue = new Queue<int>(); queue.Enqueue(1); int front = queue.Dequeue(); int peek = queue.Peek();
- Dictionaries (Hash Maps):
Dictionary<string, int> dict = new Dictionary<string, int>(); dict.Add("Alice", 25); dict["Alice"] = 26; bool exists = dict.ContainsKey("Alice");
- HashSets:
HashSet<int> set = new HashSet<int>(); set.Add(1); set.Contains(1); set.Remove(1);
- Linked Lists:
LinkedList<int> list = new LinkedList<int>(); list.AddLast(1); list.AddFirst(0); LinkedListNode<int> node = list.Find(1);
- Tuples:
var tuple = (1, "Alice"); int id = tuple.Item1; string name = tuple.Item2;
3. Algorithms
- Sorting:
Array.Sort(arr); list.Sort();
- Binary Search:
int index = Array.BinarySearch(arr, target);
- Two Pointers:
int left = 0, right = arr.Length - 1; while (left < right) { if (arr[left] + arr[right] == target) { ... } else if (arr[left] + arr[right] < target) { left++; } else { right--; } }
- Sliding Window:
int left = 0, right = 0; while (right < arr.Length) { // Expand window right++; // Shrink window if condition is met while (condition) { left++; } }
- Depth-First Search (DFS):
void DFS(Node node) { if (node == null) return; // Process node foreach (var child in node.Children) { DFS(child); } }
- Breadth-First Search (BFS):
void BFS(Node root) { Queue<Node> queue = new Queue<Node>(); queue.Enqueue(root); while (queue.Count > 0) { Node node = queue.Dequeue(); // Process node foreach (var child in node.Children) { queue.Enqueue(child); } } }
- Dynamic Programming (DP):
int[] dp = new int[n + 1]; dp[0] = 0; for (int i = 1; i <= n; i++) { dp[i] = dp[i - 1] + ...; }
4. Advanced Concepts
- Recursion:
int Factorial(int n) { if (n == 0) return 1; return n * Factorial(n - 1); }
- Backtracking:
void Backtrack(List<int> path, List<int> choices) { if (IsSolution(path)) { // Process solution return; } foreach (var choice in choices) { path.Add(choice); Backtrack(path, choices); path.RemoveAt(path.Count - 1); } }
- Bit Manipulation:
int a = 5; // 0101 int b = 3; // 0011 int and = a & b; // 0001 int or = a | b; // 0111 int xor = a ^ b; // 0110 int shiftLeft = a << 1; // 1010 int shiftRight = a >> 1; // 0010
5. Popular Libraries and Functions
- LINQ (Language Integrated Query):
var filteredList = list.Where(x => x > 0).ToList(); var sortedList = list.OrderBy(x => x).ToList(); var sum = list.Sum(); var max = list.Max();
- Math Functions:
int max = Math.Max(a, b); int min = Math.Min(a, b); double sqrt = Math.Sqrt(x); double pow = Math.Pow(x, 2);
- String Manipulation:
string s = "Hello"; string sub = s.Substring(1, 3); // "ell" string[] parts = s.Split(' '); string trimmed = s.Trim(); bool contains = s.Contains("ell");
- DateTime:
DateTime now = DateTime.Now; DateTime date = new DateTime(2023, 10, 1); TimeSpan diff = date2 - date1;
6. Tips for LeetCode Problems
- Understand the Problem: Read the problem statement carefully and identify input/output constraints.
- Choose the Right Data Structure: Use arrays, lists, dictionaries, or other structures based on the problem.
- Optimize Time and Space Complexity: Aim for the most efficient solution (e.g., O(n) or O(log n)).
- Test Edge Cases: Consider empty inputs, large inputs, and edge cases.
- Practice Regularly: Solve problems daily to improve your skills.
By mastering these concepts and practicing regularly, you'll be well-prepared to tackle LeetCode problems in C#. Good luck!